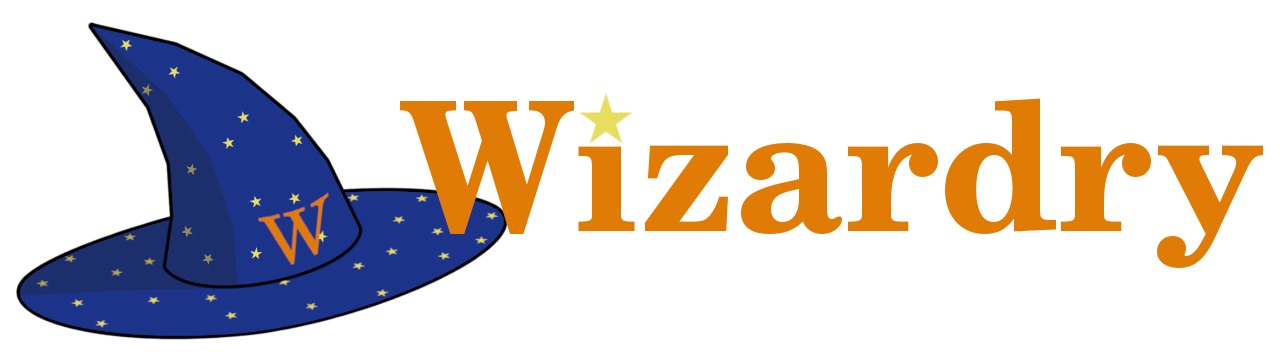
About
Wizardry is a system programming language with a C-like syntax and some extra features.These features include:
-
:defer
allows you to add code to run before exiting the scope.defer
You can add a single statement or a whole block enclosed in curly brackets.
It can be useful in the case you want to allocate multiple variables but need to check for things along the way that may cause a return.
-
All-in-one types:
will declareint [5 ]$ [2 ]myvar ;
as an array of five pointers to arrays of 2 integers.myvar -
An improved
andprintf
syntax:scanf
-
Function overloading and non-invasive symbol mangling:
Links
Documentation:Example code
A hello world program:}
A hypothetical function showing a possible use for the
defer
keyword:}
A program that displays info about the arguments passed to it:
}